Wednesday, June 18, 2008
Working with arrays in ActionScript3
This method proves to be good until we need to store four to five variables. Think of the situation where we need to store ten to twenty values. Storing in variable will increase the length of the program also it is difficult to remember all the variable names. In such situations arrays come to rescue. An array is data structure where we can store multiple values. Each value is given a unique non-negative index number. This index number starts with 0 and increments by 1 for each subsequent vale in the array.
Thursday, May 29, 2008
Using StartDrag Method In ActionScript 3
The following example demonstrates the use of startDrag method
import flash.display.Sprite
import flash.events.MouseEvent
var circle:Sprite=new Sprite();
circle.graphics.beginFill(0xFFCC00);
circle.graphics.drawCircle(225,225,20);
addChild(circle);
circle.addEventListener(MouseEvent.MOUSE_DOWN,dragmethod);
function dragmethod(Event:MouseEvent):void{
circle.startDrag();
}
The first line imports the class Sprite from the disply package. The second line then imports
the mouseEvent class from event package. Then we create circle sprite.
We have use the graphics property of the sprite in the next line to draw and fill the circle .
The beginFill graphic method takes octal color value as an argument and the draw circle takes three arguments which are x postion, y postion and the radius of circle respectively.
Then we add this circle sprite on the stage by using the addChild method.
The addEventListner method is used to register the listner function(dragmethod)
with the target of the event(circle sprite). we can name this listnerfunction withany valid identifier.
Then we create the listner function(dragmethod) which contains the statement circle.startDrag()
which makes the circle draggable when user clicks the circle.
Wednesday, May 28, 2008
Sprite in ActionScript 3
Example:
Import flash.display.Sprite
var circle:Sprite=new Sprite();
circle.graphics.beginFill(0xFFCC00);
circle.graphics.drawCircle(200,200,20);
addChild(circle);
In the above example we have imported the class Sprite. In the next line we have declared a new class circle. By using the graphics property we draw a circle of radius 20 at the location 200 & 200. We fill this circle by the yellow color using the beginfill() Method. Finally, the addChild method adds circle instance on the stage.
Tuesday, May 27, 2008
Package in ActionScript 3
Package{
Class classname
{
…………
}
}
Package allows grouping of the code so that it can be imported by some other script. We use Package keyword to show that class belongs to that package.
The Flash Player API classes are in the flash.* package. For example Flash API contains package flash.net package for transmitting data to and from the server. The package flash.* contains the following classes:
1) The action Script Component Classes which are included in the Packages: fl.accessibility, fl.conatiners, fl.controls, fl.core, fl.data, fl.events, fl.livepreview and fl.managers.
2) FLV playback component classes are included in the Package fla.video
3) Local class for Multilanguage classes are in the Package: fl.lang
4) The Motion classes are included in the Package: fl.motion
5) The Tween and Transition classes are included in the Package: fl.transitions
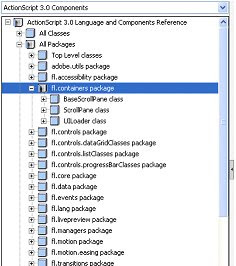
Containers Package contains ScrollPane Class
Wednesday, May 7, 2008
Difference In Event Handling Between ActionScript 2 and ActionScript 3
To overcome these problem Single Event Handling system is developed which is based on DOM (Document Object Model) level 3 specifications.
Tuesday, May 6, 2008
Object In Action Script 3
- Properties
- Methods
- Events
Properties are the group of data binded togather with object. As explained above a flower object might have the properties like smell, color, shape etc. Similarly the movie clip class has the properties like rotation, alpha, scale etc. In the following example the code scales the movie clip fly to the double of its existing size.
fly.scaleY=2;
The syntax can be: object.property=value
This whole structure is taken as a single variable and stored in a memory as a single value.
Method is an action that instructs object to do a perticular task. Suppose we have a movieclip named fly which has its separate timeline and contains some animation on it. Then we can instruct this movie clip to play by using the following code:
fly.play() where the fly is a movie clip object and play is the method.
As we know that Action Script is a programming language which is used with flash for creating interactive application and websites. This interaction involves event. When user click on some element we can set how it will respond on thhat event.
Compatibility of Action Script with earlier versions
- We can not combine Action Script 1.0 or 2.0 code with 3.0 code in a single SWF file.
- Action Script 3 can load SWF file that is programmed in 1.0 or 2.0 but can not access its variables or functions.
- SWF that is written by using Action Script 1.0 or 2.0 cant load SWF that is written using Action Script3.